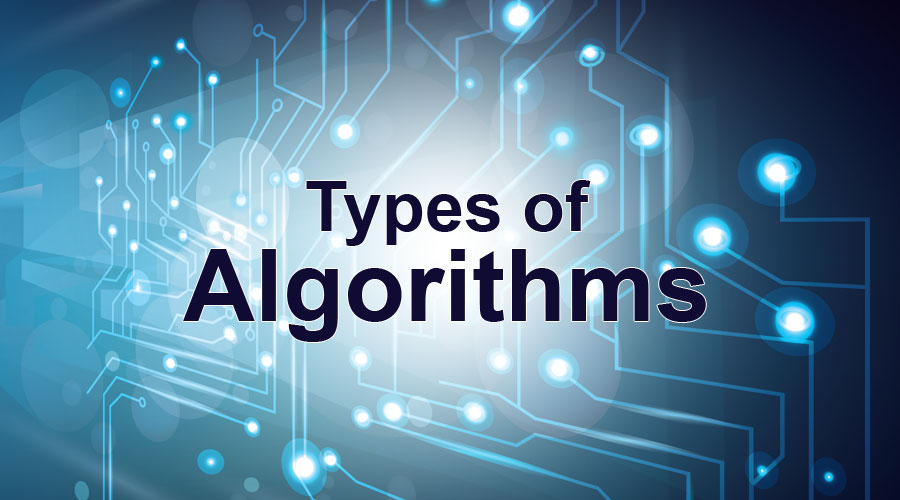
Introduction: Algorithms are everywhere around us in the digital world, from the search engines we use to the social media we interact with. But what exactly are algorithms, and how do they work? In this article, we’ll explore the basics of algorithms, their role in computer science, and how they impact our daily lives.
What are algorithms?
An algorithm is a step-by-step process for solving a problem or completing a task. It’s a set of instructions that can be followed to achieve a specific result. Algorithms can be expressed in many forms, including flowcharts, pseudocode, or actual code.
Types of algorithms
There are various types of algorithms used in computer science, some of the most common types include:
-
- Sorting algorithms: Sort data in a particular order, such as alphabetical or numerical order.
- Searching algorithms: Search for a specific value or item in a dataset.
- Graph algorithms: Analyze and manipulate graphs, which are mathematical structures used to model relationships between objects.
- Encryption algorithms: Encrypt and decrypt data, often used in secure communication systems.
How do algorithms work?
Algorithms work by breaking down a complex problem into smaller, more manageable steps. Each step is designed to move closer to the desired outcome. For example, a sorting algorithm might compare two values and swap their positions if they’re in the wrong order. This process is repeated until the entire dataset is sorted.
Advantages of algorithms
Algorithms have several advantages, including:
-
- Efficiency: Algorithms can solve complex problems quickly and accurately.
- Consistency: Algorithms always produce the same output given the same input.
- Automation: Algorithms can automate repetitive tasks, freeing up time for other important work.
Limitations of algorithms
While algorithms have many advantages, they also have limitations, including:
-
- Bias: Algorithms can be biased based on the data they’re trained on.
- Lack of creativity: Algorithms can only solve problems that they’re specifically designed to solve.
- Limited understanding: Algorithms can’t always understand the context of a problem or the nuances of human language.
Real-world examples of algorithms
Algorithms are used in countless applications, including:
-
- Search engines: Use algorithms to return relevant search results.
- Social media: Use algorithms to show users personalized content based on their interests and behavior.
- Navigation systems: Use algorithms to calculate the best route between two points.
- Online shopping: Use algorithms to recommend products based on a user’s purchase history.
Code example
Let’s take a look at a simple example of a sorting algorithm in Python:
def bubble_sort(arr):
n = len(arr)
for i in range(n):
for j in range(0, n-i-1):
if arr[j] > arr[j+1] :
arr[j], arr[j+1] = arr[j+1], arr[j]
arr = [64, 34, 25, 12, 22, 11, 90]
bubble_sort(arr)
print ("Sorted array is:")
for i in range(len(arr)):
print ("%d" %arr[i]),
This is a bubble sort algorithm, which sorts an array by repeatedly comparing adjacent elements and swapping them if they’re in the wrong order.
Conclusion
Algorithms are an essential part of computer science, enabling us to solve complex problems efficiently and accurately. They’re used in countless applications and impact our daily lives in many ways. While algorithms have many advantages, they also have limitations and must be used with caution.