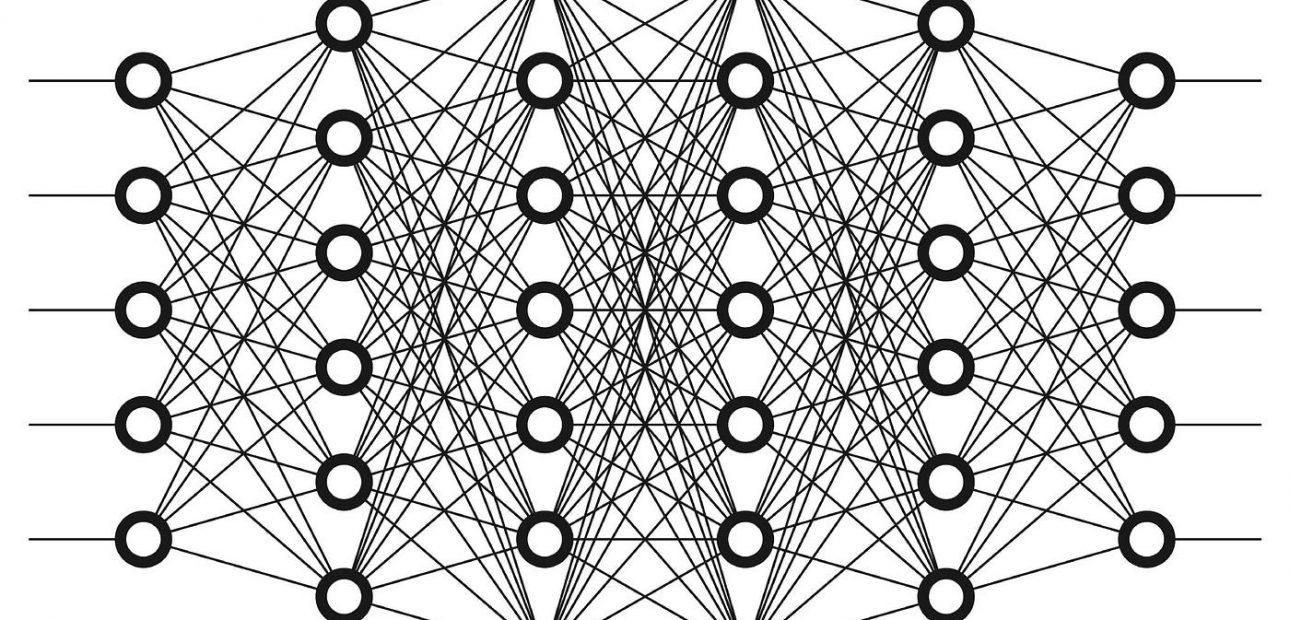
In recent years, deep learning has emerged as one of the most exciting fields of artificial intelligence. It has been used to solve a wide range of problems, from image and speech recognition to natural language processing and self-driving cars. In this article, we will explore what deep learning is, how it works, and some of its applications.
What is Deep Learning?
Deep learning is a subfield of machine learning that involves training neural networks to recognize patterns in data. A neural network is a type of machine learning model that is inspired by the structure and function of the human brain. It is composed of layers of interconnected nodes, each of which performs a simple mathematical operation.
The term “deep” in deep learning refers to the fact that these neural networks can have many layers, which allows them to learn complex patterns in data. Deep learning algorithms are typically trained on large datasets, which enables them to identify subtle patterns that may be difficult or impossible for humans to detect.
How Does Deep Learning Work?
Deep learning involves a multi-step process that begins with data preparation and ends with the deployment of a trained model. Here is a brief overview of each step:
-
Data Preparation: This step involves cleaning and formatting the data, as well as splitting it into training, validation, and testing sets.
-
Model Definition: In this step, the neural network architecture is defined, including the number of layers, the types of nodes used in each layer, and the activation functions used to transform the input data.
-
Training: During the training phase, the neural network is fed the training data, and the weights of the connections between the nodes are adjusted in response to the errors made by the model. This process is repeated multiple times until the model reaches a certain level of accuracy.
-
Validation: The validation set is used to evaluate the performance of the model during the training phase. It is used to prevent overfitting, which occurs when the model performs well on the training data but poorly on new data.
-
Testing: The testing set is used to evaluate the final performance of the trained model. It is used to ensure that the model can generalize to new data and perform well in real-world scenarios.
Applications of Deep Learning
Deep learning has been used to solve a wide range of problems across many industries. Here are a few examples:
- Image Recognition: Deep learning has been used to develop highly accurate image recognition systems that can identify objects and people in images and videos.
Code Example:
import tensorflow as tf
from tensorflow import keras
# Load the dataset
(train_images, train_labels), (test_images, test_labels) = keras.datasets.mnist.load_data()
# Normalize the images
train_images = train_images / 255.0
test_images = test_images / 255.0
# Define the model architecture
model = keras.Sequential([
keras.layers.Flatten(input_shape=(28, 28)),
keras.layers.Dense(128, activation='relu'),
keras.layers.Dense(10)
])
# Compile the model
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=10)
# Evaluate the model
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print('\nTest accuracy:', test_acc)
-
Natural Language Processing: Deep learning has been used to develop language models that can understand and generate human language. These models are used in virtual assistants, chatbots, and translation software.
-
Self-Driving Cars: Deep learning has been used to develop self-driving car systems that can recognize and respond to objects in their environment This technology has the potential to greatly reduce the number of accidents caused by human error.
- Healthcare: Deep learning has been used to analyze medical images and data, which can help doctors diagnose diseases and develop personalized treatment plans.
Code Example:
import tensorflow as tf
from tensorflow import keras
# Load the dataset
(train_images, train_labels), (test_images, test_labels) = keras.datasets.cifar10.load_data()
# Normalize the images
train_images = train_images / 255.0
test_images = test_images / 255.0
# Define the model architecture
model = keras.Sequential([
keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
keras.layers.MaxPooling2D((2, 2)),
keras.layers.Conv2D(64, (3, 3), activation='relu'),
keras.layers.MaxPooling2D((2, 2)),
keras.layers.Conv2D(64, (3, 3), activation='relu'),
keras.layers.Flatten(),
keras.layers.Dense(64, activation='relu'),
keras.layers.Dense(10)
])
# Compile the model
model.compile(optimizer='adam',
loss=tf.keras.losses.SparseCategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
# Train the model
model.fit(train_images, train_labels, epochs=10)
# Evaluate the model
test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2)
print('\nTest accuracy:', test_acc)
Common Deep Learning Techniques
There are many techniques that are commonly used in deep learning, including:
-
Convolutional Neural Networks (CNNs): These networks are designed for image recognition and processing tasks. They use a type of node called a convolutional layer, which can identify patterns in small regions of an image.
-
Recurrent Neural Networks (RNNs): These networks are designed for processing sequential data, such as natural language or time-series data. They use a type of node called a recurrent layer, which can remember information from previous time steps.
-
Generative Adversarial Networks (GANs): These networks are designed for generating new data that is similar to a training dataset. They consist of two neural networks, a generator and a discriminator, which are trained together in a competition-like fashion.
-
Transfer Learning: This technique involves using a pre-trained deep learning model as a starting point for a new task. This can greatly reduce the amount of training data required for the new task and speed up the training process.
Frequently Asked Questions
Q: What is the difference between machine learning and deep learning?
A: Machine learning is a broad field that includes many different techniques for training models to make predictions based on data. Deep learning is a subfield of machine learning that specifically involves training neural networks to recognize patterns in data.
Q: What are the advantages of deep learning?
A: Deep learning has several advantages over traditional machine learning techniques, including the ability to learn complex patterns in data, the ability to automatically extract features from raw data, and the ability to improve performance as more data is provided.
Q: What are some common challenges in deep learning?
A: Some common challenges in deep learning include overfitting, which occurs when the model performs well on the training data but poorly on new data, and vanishing gradients, which occurs when the gradient used to update the weights of the network becomes too small to be effective.
Conclusion
Deep learning is a rapidly evolving field that has the potential to transform many industries. By training neural networks to recognize patterns in data, deep learning algorithms can help solve complex problems in image recognition, natural language processing, self-driving cars, healthcare, and other fields. With the ability to automatically extract features from raw data, deep learning algorithms can also reduce the amount of manual feature engineering required in traditional machine learning.
While deep learning has many advantages, it also presents several challenges, such as overfitting and vanishing gradients. However, researchers are continually developing new techniques to overcome these challenges and improve the performance of deep learning models.
Overall, deep learning is a powerful tool for solving complex problems and has the potential to revolutionize many industries in the years to come.